It is super easy to get started with gRPC on .NET. Most of the heavy lifting is done by Visual Studio
Create the project
First, open Visual Studio and select the ASP.NET Core gRPC Service project template
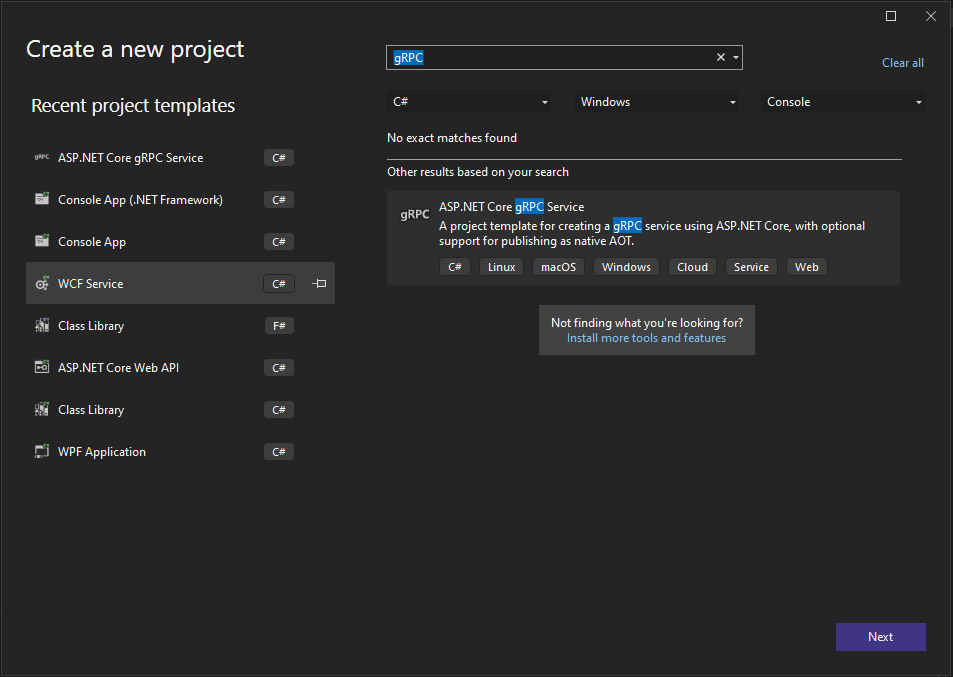
then add the name of the project and click Next. Follow the wizard up to completion.
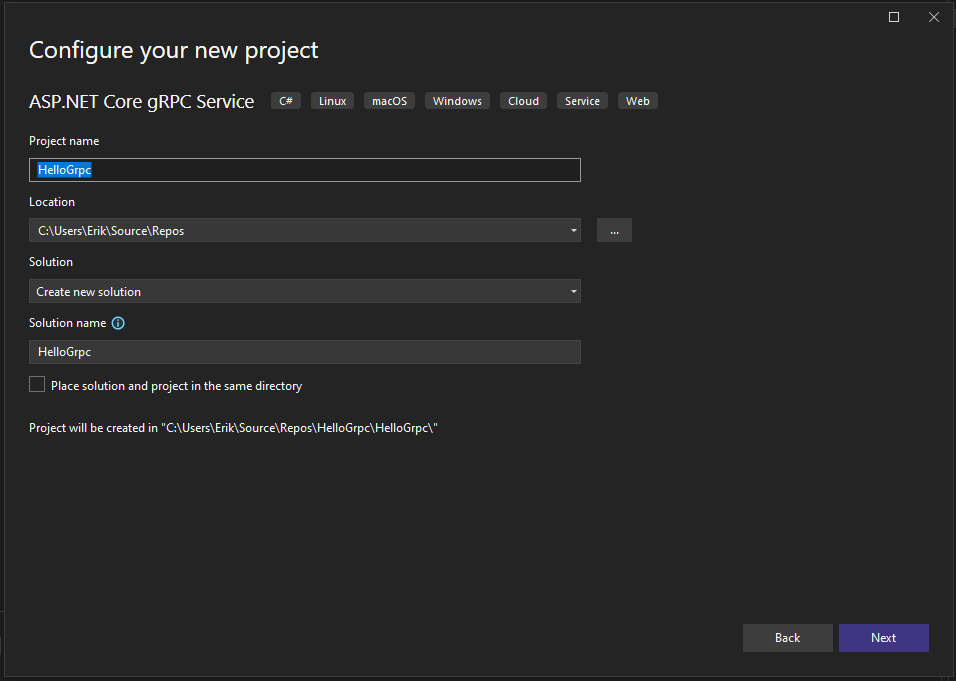
(Optional) Enable server reflection
After Visual Studio has created the project files, right-click on Dependencies then select Manage Nuget Packages.
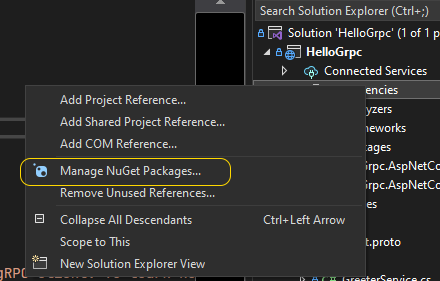
Search for Grpc.AspNetCore.Server.Reflection and then click Install
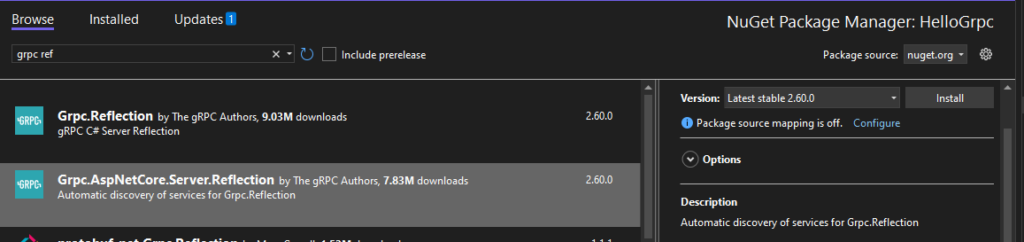
Now open up Program.cs and let’s add a couple lines of code to enable server reflection. We do this on lines 7 and 14. We’ll need these later on to test that our service is working perfectly
using HelloGrpc.Services;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddGrpc();
builder.Services.AddGrpcReflection();
var app = builder.Build();
// Configure the HTTP request pipeline.
app.MapGrpcService<GreeterService>();
app.MapGrpcReflectionService();
app.MapGet("/", () => "Communication with gRPC endpoints must be made through a gRPC client. To learn how to create a client, visit: https://go.microsoft.com/fwlink/?linkid=2086909");
app.Run();
Run the gRPC service
Now, hit F5 (or click on the green Debug button) to run the services. Visual Studio will compile the project and then run it. As can be seen from the screenshot below, the service is hosted at port 7059 with an https endpoint and at 5013 for http
(Note: the port numbers will be different for your own machine)
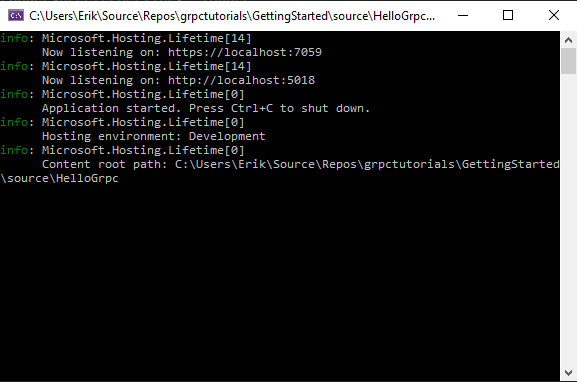
That’s it! Your first gRPC Service is now up and running.
Test the gRPC service
Now let’s try to test it to see whether it can respond to http calls. For this we will need a test client. A great tool, and one that that I personally use, is FintX (https://github.com/namigop/FintX/). It’s an open-source, native and cross-platform gRPC client. I’m on Windows, so I have installed the windows package (macOS and Linux downloads are also available)
To add the our gRPC Service to FintX, select Reflection Service (this is why in the previous steps we enabled the reflection service) and add the other required fields.
Click Okay.
FintX will automatically generate the client code (C#) and compile it. Once, it is done it will display the gRPC methods in a treeview
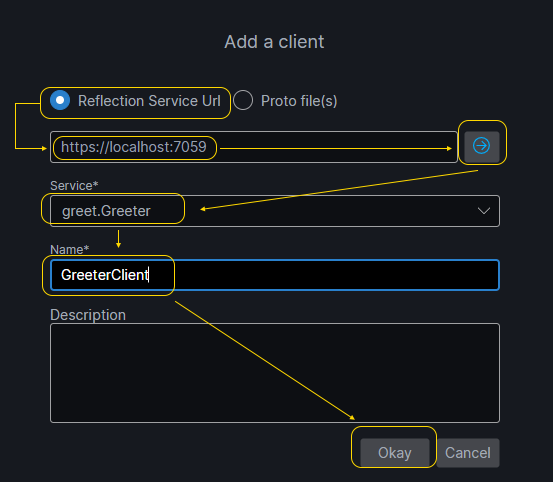
Now, let’s invoke our gRPC service. Double-click the method “SayHello” or click on the open tab button. In the request editor, enter a name then click on the green Run button in the middle – FintX will now call our gRPC service.
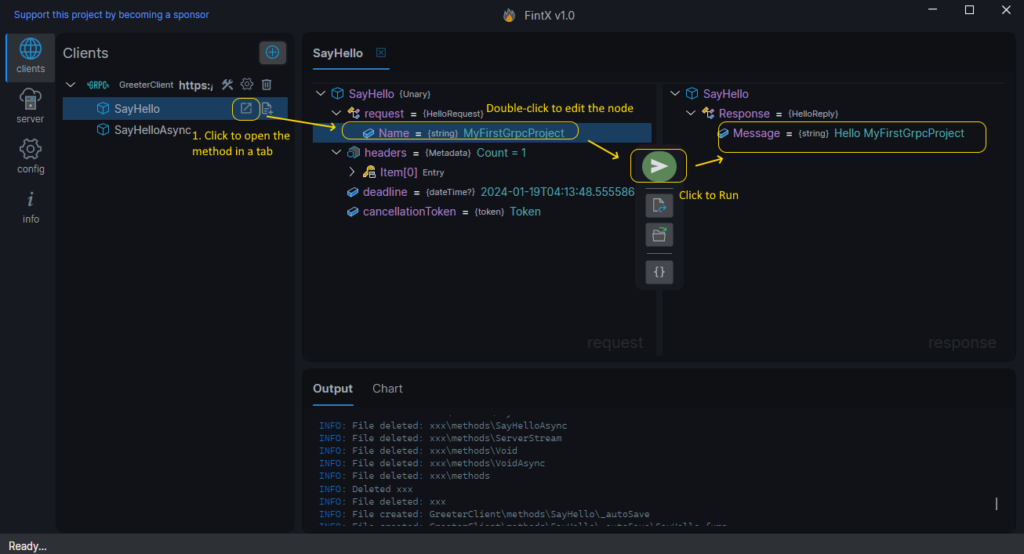
And there you go, as can be seen from the screenshot below our service is working perfectly. We can see that it has sent back a response with the Name parameter that we passed in the request
Others
The full source code of this tutorial can be downloaded from https://github.com/namigop/grpctutorials/tree/main/GettingStarted. Happy coding!
Pingback: C#/.NET gRPC Service with Server Streaming – gRPC Tutorials